ESP32Cam has always been implicitly recommended by makers, since you get a builtin cam for quite a cheap price. Most have come across a situation where they have to use I2C Bus for interfacing I2C sensors with the ESP32Cam. There won't be any I2C Pins when you look at the pinout since the Omnivision Camera Sensor is internally connected to the I2C Pins of ESP32. But there are two I2C Bus in an ESP32 and you can literally use any pins as Data Line and Clock Line. Below is an example of an I2C sensor interfaced with an ESP32Cam.
Dependencies
- Adafruit BME280
- Adafruit BusIO
- Adafruit Unified Sensors
Note : Used Adafruit BME (2.1.1), Adafruit BusIO (1.4.1) & Adafruit Unified Sensors (1.1.4) Libraries. Everything including the required ibraries is added at the last section. Refer the error section in this page before you use the libraries from Adafruit Repositories.
BME280 Sensor - I2C Bus Example
#include "Arduino.h"
#include <Wire.h>
// #include "esp_camera.h"
#include <Adafruit_Sensor.h>
#include <Adafruit_BME280.h>
// -----------------I2C-----------------
#define I2C_SDA 14 // SDA Connected to GPIO 14
#define I2C_SCL 15 // SCL Connected to GPIO 15
TwoWire I2CSensors = TwoWire(0);
// BME 280 (Using I2C)
Adafruit_BME280 bme;
// Sensor Variable (BME280)
float temperature, humidity;
void setup()
{
Serial.begin(115200);
I2CSensors.begin(I2C_SDA, I2C_SCL, 100000);
// BME 280 (0x77 or 0x76 will be the address)
if (!bme.begin(0x76, &I2CSensors))
{
Serial.println("Couldn't Find BME280 Sensor");
while(1);
}
else
{
Serial.println("BME280 Sensor Found");
}
}
void loop()
{
// -------------Temperature (C)------------------
temperature = bme.readTemperature();
Serial.print("Temperature = ");
Serial.print(temperature);
Serial.print(" *C - ");
// ----------------------------------------------
// ---------------Humidity (%)-------------------
humidity = bme.readHumidity();
Serial.print("Humidity = ");
Serial.print(humidity);
Serial.println(" %");
// ----------------------------------------------
delay(1000);
}
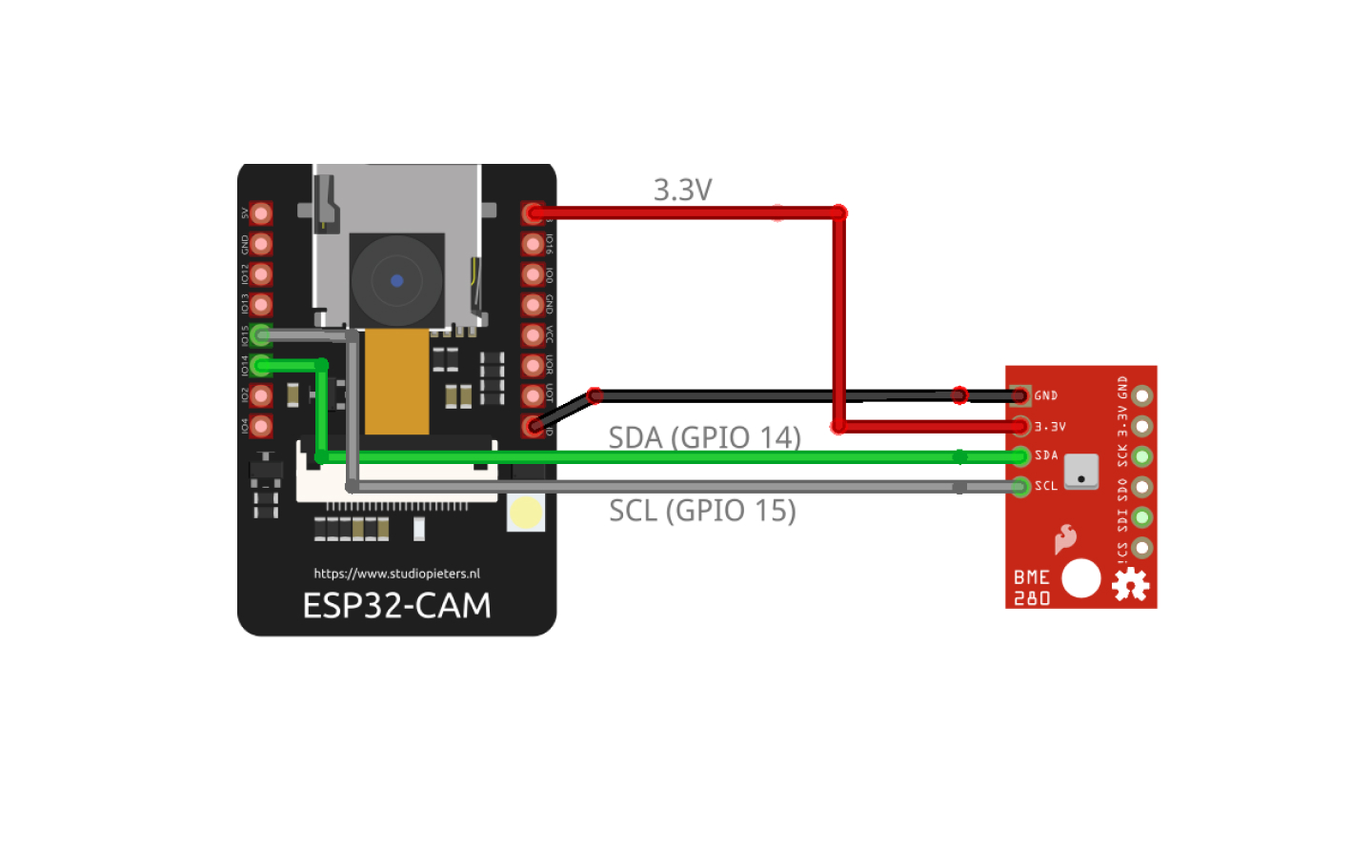
I2C Bus
-
Define SDA and SCL Pins, Pin Number 14 and 15 are selected respectively.
#define I2C_SDA 14 #define I2C_SCL 15
-
Create a Two Wire Instance.
TwoWire I2CSensors = TwoWire(0);
-
In setup(), intialize the Two Wire Instance by passing in the SDA & SCL Pins and the clock frequency.
I2CSensors.begin(I2C_SDA, I2C_SCL, 100000);
BME280 Sensor
-
Create an instance of the sensor using the Adafruit BME280 Library.
Adafruit_BME280 bme;
-
Initalize the Sensor by passing in the I2C Address of BME280 and the configured TwoWire Instance.
if (!bme.begin(0x76, &I2CSensors)) { Serial.println("Couldn't Find BME280 Sensor"); while(1); } else { Serial.println("BME280 Sensor Found"); }
Expected Error (Adafruit Library)
An expected error while using Adafruit Libraries is that sensor_t
will be conflicting with the ESP32Cam Board since it is declared both in Adafruit Library and ESP32Cam Board Library. This happens only when you include "esp_camera.h", that is if you use camera.
In file included from src/main.cpp:4:0:
lib/Adafruit_Unified_Sensor/Adafruit_Sensor.h:155:3: error: conflicting declaration 'typedef struct sensor_t sensor_t'
} sensor_t;
^
In file included from /home/abish/.platformio/packages/framework-arduinoespressif32/tools/sdk/include/esp32-camera/esp_camera.h:70:0,
from src/main.cpp:2:
/home/abish/.platformio/packages/framework-arduinoespressif32/tools/sdk/include/esp32-camera/sensor.h:133:3: note: previous declaration as 'typedef struct _sensor sensor_t'
} sensor_t;
^
*** [.pio/build/esp32cam/src/main.cpp.o] Error 1
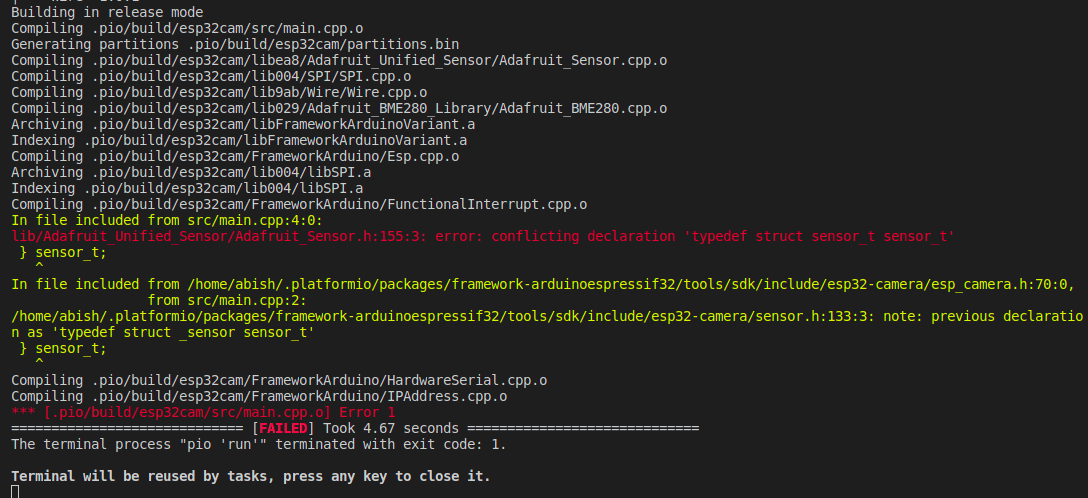
Inorder to fix this, rename all the sensor_t
instances to another name, for example sensor_t1
. I used Sublime Text for renaming all this at once. Also I have attached the modified libraries. Rename yourself if you want to use the latest version of the library when it releases.
Github Repository (Including Libraries). Comment down below if you have any queries.